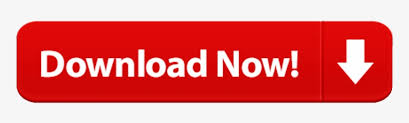
Our version works in reverse from the animation, but it’s close enough. This way all of the largest values build up, or “bubbles up”, on the end. In selection sort, the minimum element is selected from the array and swap with an element which is at the beginning of the unsorted sub array. If the adjacent elements are not at the correct position, swapping would be performed. To avoid recomparing sorted numbers we’ll start from the back of the array while another for loop gets the preceding number. In bubble sort, two adjacent elements are compared. This is the “Hello World” of sorting methods, nothing crazy but it gets the job done.įor each item in the array we want to check if the next item is larger, if it is then swap their indexes in the array. I’m going to be looking at everything through the lens of Big O Notation, so understanding how complexity grows over time will be very helpful. Both algorithms have a time complexity of. On the contrary, on each iteration, the bubble sort algorithm compares and swaps the adjacent elements.

Yet, on th iteration, the insertion sort algorithm compares the th element against the first elements. Here’s the practice array of 50 random numbers. Both of the algorithms compare the elements to find their order. Keep in mind that these are more general concepts and patterns and less of a “how-to” for sorting data since your particular implementation may differ a lot but in the end it may conceptually resemble these patterns. integers, floating-point numbers, strings, etc) of an array (or a list) in. It uses item exchanging to swap elements. It is slower in comparison to selection sort. It is efficient in comparison to selection sort. Based on the adjacent elements, swaps are made. Whether your data is sorted or not will directly affect what search methods you can use and can mean the difference between a search taking a million operations or taking 10, like with Binary Search.įor simplicity’s sake, we’re only going to focus on sorting an array of numbers from least to greatest, but these algorithms are easily modifiable for other sorting goals. Sorting is a very classic problem of reordering items (that can be compared, e.g. It iterates through the list, and compares adjacent pairs of elements to sort them. Third Iteration: Start the following Iteration with the fourth element (13), and compare it with its preceding elements.įollowing are C, C++, Java and Python implementations of Insertion Sort.Having your datasets arranged all willy-nilly will only add more time and resources to manage and search through. The array after the Second iteration looks like: Inserstion Sort - this one is actually pretty good on nearly sorted collections. The comparison shows 17 25, no swapping takes place.Īlso, 31> 17, no swapping takes place and 31 remains at its position. Answer (1 of 7): Bubble Sort - pretty much only when you are teaching someone about sorting and wish to give an example of a quadratic algorithm that shouldnt really be used in practice. Let us now understand working with the following example:Ĭonsider the following array: 25, 17, 31, 13, 2įirst Iteration: Compare 25 with 17. But then the space complexity increases from O(1) to O(N), where N is the size of the array. The selection sort can be made stable by incorporating the indices of equal elements when comparing and sorting them. begingroup There are lots of sorting algorithms, so its not really surprising that you havent found a pre-packaged explanation of the similarities between two particular not-very-good algorithms. The above procedure is repeated until all the element in the array is at their apt position. Bubble sort and insertion sort are stable, whereas selection sort isn’t.


Although it has the same complexity, the inser-tion sort is a little over twice as efficient as the bubble sort. Insertion Sort: Like bubble sort, the insertion sort has a complexity of.
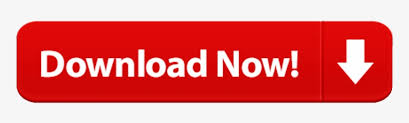